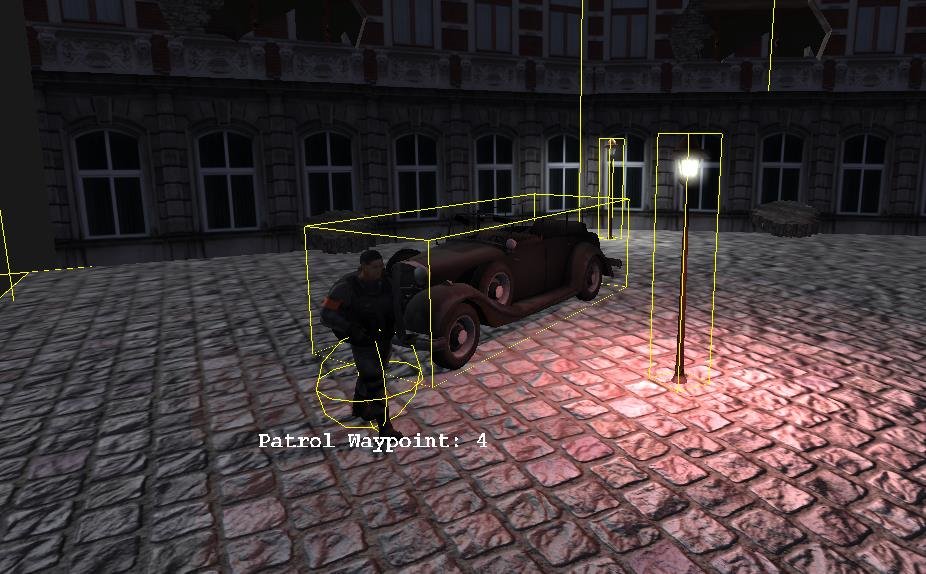
Prime Engine
A 3D Game Engine written in C++, built by Artem Kovalovs from Naughty Dog Games.
Game Engine Development
is a class I am taking with Naughty Dog’s Principal Graphics & Game Programmer Artem Kovalovs.
Prime Engine is a bare-bones 3D game engine Artem has written in his early years of game engineering. It has basic asset loading, object tracking, event systems, and rendering capabilities but not much more. It is a good starting point to learn the fundamentals of game engine development and allows me to build useful features on top of it. It also challenges my code reading capabilities as there are many custom data types, many layers of logic, and not a lot of documentation or comments.
My main achievement so far:
Understand and get comfortable with a large existing code base in C++ that is not the most well-documented or commented.
Implement Frustum Culling along with AABB bounding box that optimizes performance by not loading off-screen meshes into the renderer.
Design and build a physics engine that performs collision detection between AABB and spherical colliders while also supporting gravity.
Engineer a vertex shader that supports UV displacement to have shader-based wind physics.
Feature Highlights
Frustum Culling
By comparing the extremes of each of the meshes of the game objects with the camera’s frustum planes, we can deduce which object will be off-screen and therefore don’t need to be rendered.
While the math and logic of this technique are straightforward, I learned some valuable insights into debugging 3D applications. Spending a little more time to implement debug lines can help a lot down the line when problems arise.
When writing the comparison between the frustum planes and AABB, my first iteration would have all the objects culled out. After many struggles, I decided to draw out my far clip plane and debug each plane comparison one by one. It turned out that the normals for my far plane and near plane were flipped. Essentially, I was asking if a point was both further than the furthest plane and closer than the closest plane, a condition impossible to fulfill.
Physics System
Implementing a physics system in a 3D game engine was one of the more challenging and interesting technical tasks I have done.
Prime engine started out with no physics at all, and a character would just walk straight through a static object when instructed to. I started by creating a new physics component that is attached to every mesh-having game object in the engine. Then I made a physics manager that holds references to all static and dynamic physics objects and performs collision detection between them.
The math and logic for collision detection were trickier frustum culling. When an object intends to move to a new position, its’ physics component gets moved first and tests for collision against other objects. If no collision was detected, the object moves to its new position and the next cycle begins. Otherwise, we need to modify the direction we want to move so we can stay out of the other object’s collider.
Some interesting problems arose during development. When doing collision detection, we need to know the min and max vertices of the AABBs. However, the minX coordinate in model space may no longer be minX of that object in world space, as the object may have been rotated. Another vertex may actually be smaller in X in world space. The solution would simply be doing collision detection in the model space of each AABB. This was one of those problems that require a solid understanding of 3D math and transformation matrices to notice, and once understood, the solution was simple.
Shader Based Wind
Shader Based Wind displaces the static meshes’ vertices as if the wind blows them. The task significantly strengthened my skills in exploring a complex code base and my understanding of game engine architecture.
I added two types of wind sources, directional and point wind. I then attached the point wind source to NPCs walking in the scene, and the directional wind to blow in the camera's direction. I then pass the wind sources’ information to the vertex shader.
To make the wind displacement look more natural, I scale the vertex displacement with the y value of the vertices. The end result is that the root of the models will stay in place while the higher portions will be affected by the wind.
Character Locomotion
I set out to create an animated 3rd person character locomotion system. My main goals are to have a decent character controller that has movement, and animation and supports collision detection
I started by cleaning up the animation sets and changed the root motion animations into in-place ones. Then, I coded a 3rd-person camera to follow the player and spectate from different angles. I then moved on to code the character’s movement and turnings and hook movement states to appropriate animation states.
I changed my physics implementation so that collision sliding can happen optionally and is compatible with walking up sloped surfaces. I also separated ground collision into a separate, more efficient algorithm of making a point vs surface collision test instead of a sphere vs AABB collision test. This works well in two ways. It is impossible for objects to fall through the ground plane no matter the falling speed now, and I increased the fps for my test scene from 40~fps to a steady 65~fps.
I added jumping by using a counter to keep track of information across frames to change animation and movement state. I then added cover taking which allows the character to hide behind any static object it is in contact with and can move left or right along it. I also coded and animated states for transitioning between standing and taking cover, as well as separate movement, states for taking cover.